C++ Polymorphism
The term Polymorphism get derived from the Greek word where poly + morphos where poly means many and morphos means forms.
In programming background, polymorphism can be broadly divided into two parts. These are:
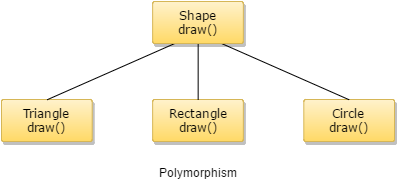
Polymorphism is another concept of object oriented programming (OOPs). The attitude which lies beneath this concept is “single interface having multiple implementations.” This provides a single interface for controlling access to a general class of actions. Polymorphism can be gained in both ways:
A common and simple example of polymorphism is when you used >> and << as operator overloading in C++, for cin and cout statements respectively. This bitwise shift operator at that time acts as a inclusion operator and its overloaded meaning is defined in iostream header file.
Static Polymorphism
In static polymorphism or early binding, there you will get two sub categories like:
Code Snippet for Function Overloading
class funcOl {
public:
funcOl ();
funcOl (int i);
int add(int a, int b);
int add(float a, float b);
};
Code snippet for Operator Overloading
class calc {
public:
// + operator overloading technique
int operator+(calc g);
private:
int k;
};
It is to be noted that function overloading can be done and is possible only based on:
Dynamic Polymorphism
This refers to the entity which changes its form depending on circumstances at run time. This concept can be adopted as analogous to a chameleon changing its color at the sight of an approaching object.
What is a Virtual Function?
A virtual function can be defined as the member function within a base class which you expect to redefine in derived classes. For creating a virtual function, you have to precede your function’s declaration within the base class with a virtual keyword.
Example of how to use virtual function
Example:
#include <iostream> using namespace std; class Game { int g; public: Game() { g = 1; } virtual void show() { cout <<g; } }; class Anim: public Game { int k; public: Anim() { k = 2; } virtual void show() { cout <<k; } }; int main() { Game *g; Anim a; g = &a; g->show(); return 0; }
No comments:
Post a Comment