Pointer is a variable that points to an address of a value.
Pointer => address that contains the value
Symbols used in pointer :
& (ampersand sign) : ‘Address of operator’. It determines the address of a variable.
* (asterisk sign) : indirection operator / value at address. Accesses the value at the address.
Example :
int i = 3 ;
This declaration tells the C compiler to :-
- Reserve space in memory to hold the integer value.
- Associate the name i with this memory location.
- Store the value 3 at this location.
We may represent the location of i in the memory by :-
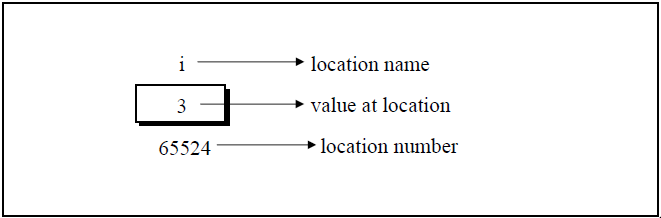
- The computer has selected memory location 65524 as the place to store the value 3.
- The location number 65524 is not a number to be relied upon, because some other time the computer may choose a different location for storing the value 3.
- The important point is, i’s address in memory is a number.
Example :
#include <stdio.h>
#include <conio.h>
void main()
{
int i = 3 ;
printf ( "\nAddress of i = %u", &i);
printf ( "\nValue of i = %d", i);
printf ( "\nValue of i = %d", *(&i));
}
Output :
Address of i = 65524 Value of i = 3
Explanation :
- The pointer points to the address 65524 of variable i, that contains the value of i i.e 3
- Pointer points to ->65524 address that points to =>value 3
- &i gives the address of the variable i.
- *i gives the value store at the address of i.
- ‘&’ => ‘address of ’ operator.
- The expression &i in the first printf() statement returns the address of the variable i, which in this case happens to be 65524.
- As 65524 is an address, there is no sign associated with it. So to print the address we use ‘%u’ which is a format specifier for printing an unsigned integer.
- The another pointer operator is ‘*’ called ‘value at address’ operator which gives the value stored at a particular address.
- The ‘value at address’ operator is also called ‘indirection’ operator.
- *(&i) is same as printing the value of i.
ReplyDeleteExcellent blog on testing tool. Looking for software courses?
Qtp training in Chennai
iOS Training in Chennai
JAVA Training in Chennai
Big Data Training in Chennai
Selenium Training in Chennai
German Classes in chennai
French Classes in Chennai
french coaching classes in chennai
French Classes in Anna Nagar
Informative post indeed, I’ve being in and out reading posts regularly and I see alot of engaging people sharing things and majority of the shared information is very valuable and so, here’s my fine read.
ReplyDeleteclick here button gif
click here book
click here button image
click here button html
click here blinking button